Simple One-Ventricle Closed Circulation
Todo
Make One-Ventricle Circulation figure
(Source code, png, hires.png, pdf)
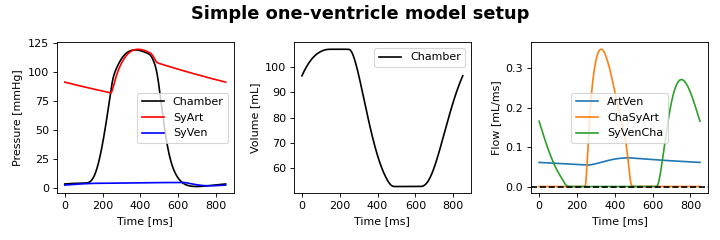
The full code to generate this plot is shown below.
python
1# -*- coding: utf-8 -*-
2import sys
3
4# check if should include path for development modus
5# try:
6# __import__('circadapt')
7# except:
8# sys.path.append('../../../src/')
9
10# actual import
11from circadapt import CircAdapt
12import numpy as np
13import matplotlib.pyplot as plt
14
15import time
16
17def create_model():
18 ###
19 n_beat = 10
20
21 dT = 0.001
22 solver = "SolverFE"
23
24 # open model
25 model = CircAdapt("Custom",
26 solver,
27 )
28
29 model.set('Solver.dT', dT)
30 model.set('Solver.dTexport', dT)
31
32 # Build the cavity C using a Chamber2022 object. This object automatically
33 # gets an Wall2022 object with no patches, so a patch must be added.
34 model.add_component('Chamber2022', 'C')
35 model.add_component('Patch2022', 'P', 'C.wC')
36
37 # Use 'smart component' function to add an ArtVen combination.
38 model.add_smart_component('ArtVen')
39
40 # Add and connect valves for inflow and outflow of the cavity
41 model.add_component('Valve2022', 'ChaSyArt')
42 model.add_component('Valve2022', 'SyVenCha')
43
44 model.set_component('ChaSyArt.Prox', 'C')
45 model.set_component('ChaSyArt.Dist', 'SyArt')
46 model.set_component('SyVenCha.Prox', 'SyVen')
47 model.set_component('SyVenCha.Dist', 'C')
48
49 # set state variables
50 model.set('Model.C.V', 125e-6)
51 model.set('Model.SyArt.V', 200e-6)
52 model.set('Model.SyVen.V', 300e-6)
53
54 # parameterize patch
55 model['Patch2022']['dT'] = 0.1
56 model['Patch2022']['SfAct'] = 200e3
57 model['Patch2022']['SfPas'] = 5e3
58 model['Patch2022']['AmRef'] = 0.014
59 model['Patch2022']['k1'] = 10
60 model['Patch2022']['vMax'] = 7
61 model['Patch2022']['VWall'] = 1e-04
62 # model['Patch2022']['Lsi'] = par_Lsi
63 model['Patch2022']['TR'] = 0.25
64 model['Patch2022']['TD'] = 0.25
65 model['Patch2022']['TimeAct'] = 0.5
66 model.set('Model.C.wC.P.Lsi', 2.0)
67
68
69 model['ArtVen']['p0AV'][0] = 9000
70
71 # Run beats
72 t0 = time.time()
73 model.run(n_beat)
74 print(time.time()-t0)
75
76 return model
77
78# %% Run model and plot
79if __name__ == '__main__':
80 model = create_model()
81 plt.figure(1, clear=True, figsize=(9, 3))
82
83 t = model.get('Solver.Time') * 1e3
84
85 m=1
86 n=3
87
88 ax = plt.subplot(m,n,1)
89 ax.plot(t, model.get('Model.C.p')/133, label='Chamber', color='k')
90 ax.plot(t, model.get('Model.SyArt.p')/133, label='SyArt', color='r')
91 ax.plot(t, model.get('Model.SyVen.p')/133, label='SyVen', color='b')
92 plt.legend()
93 ax.set_ylabel('Pressure [mmHg]')
94 ax.set_xlabel('Time [ms]')
95
96 ax = plt.subplot(m,n,2)
97 ax.plot(t, model.get('Model.C.V')*1e6, label='Chamber', color='k')
98 ax.set_ylabel('Volume [mL]')
99 ax.set_xlabel('Time [ms]')
100 plt.legend()
101
102 ax = plt.subplot(m,n,3)
103 ax.plot(t, model.get('Model.CiSy.q')*1e3, label='ArtVen')
104 ax.plot(t, model.get('Model.ChaSyArt.q')*1e3, label='ChaSyArt')
105 ax.plot(t, model.get('Model.SyVenCha.q')*1e3, label='SyVenCha')
106 plt.legend()
107
108 ax.axhline(0, color='k', linestyle='--')
109 ax.set_ylabel('Flow [mL/ms]')
110 ax.set_xlabel('Time [ms]')
111 plt.legend()
112
113 plt.suptitle('Simple one-ventricle model setup', fontsize=16, weight='bold')
114
115
116 # ax = plt.subplot(m,n,4)
117 # ax.axhline(0, color='k', linestyle='--', lw=1)
118 # ax.set_ylabel('C')
119 # plt.legend()
120
121 plt.tight_layout()
122 plt.draw()
123 plt.show()
124