VanOsta2022 Regional Myocardial (Dys)function
The multipatch implementation of the wall module allows for simulationg regional wall mechanics. To initiate this, the wall can be split in multiple patches using the lines:
Running this simulation results in the following pressures, volumes, and regional fiber strain.
(Source code, png, hires.png, pdf)
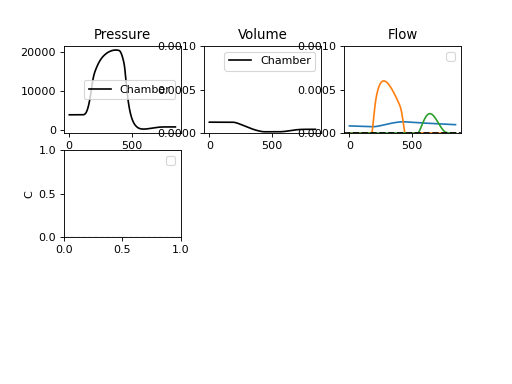
The full code to generate this plot is shown below.
python
1# -*- coding: utf-8 -*-
2import sys
3
4# check if should include path for development modus
5try:
6 __import__('circadapt')
7except:
8 sys.path.append('../../../src/')
9
10# actual import
11from circadapt import CircAdapt
12import numpy as np
13import matplotlib.pyplot as plt
14
15import time
16
17def create_model():
18 ###
19 par_SfAct = 120e3
20 par_SfAct1 = 120e3#20e3
21 par_vMax = 7.
22 par_Lsi = 1.
23
24 n_beat = 1
25
26 dT = 0.001
27 solver = "SolverFE"
28
29 # open model
30 model = CircAdapt("Custom",
31 solver,
32 "../../../../core/x64/DLL/CircAdapt Core.dll",
33 )
34
35 model.set('Solver.dT', dT)
36 model.set('Solver.dTexport', dT)
37
38 model.add_component('Chamber2022', 'C')
39 model.add_component('Patch2022', 'P', 'C.wC')
40
41 model.add_smart_component('ArtVen')
42
43 model.add_component('Valve2022', 'ChaSyArt')
44 model.add_component('Valve2022', 'SyVenCha')
45
46 model.set_component('ChaSyArt.Prox', 'C')
47 model.set_component('ChaSyArt.Dist', 'SyArt')
48 model.set_component('SyVenCha.Prox', 'SyVen')
49 model.set_component('SyVenCha.Dist', 'C')
50
51 # set state variables
52 model.set('Model.C.V', 125e-6)
53 model.set('Model.SyArt.V', 200e-6)
54 model.set('Model.SyVen.V', 300e-6)
55
56 # parameterize
57 model['Patch2022']['dT'] = 0.1
58 model['Patch2022']['SfAct'] = par_SfAct
59 model['Patch2022']['SfPas'] = 15e3
60 model['Patch2022']['AmRef'] = 1*0.009805314057621018
61 model['Patch2022']['k1'] = 10
62 model['Patch2022']['vMax'] = par_vMax
63 model['Patch2022']['VWall'] = 1*9.60080108859341e-05
64 # model['Patch2022']['Lsi'] = par_Lsi
65 model['Patch2022']['TR'] = 0.25
66 model['Patch2022']['TD'] = 0.25
67 model['Patch2022']['TimeAct'] = 0.4
68 model.set('Model.C.wC.P.Lsi', par_Lsi)
69
70 t0 = time.time()
71 model.run(n_beat)
72 print(time.time()-t0)
73
74 return model
75
76# %% Run model and plot
77if __name__ == '__main__':
78 model = create_model()
79 plt.figure(1)
80 plt.clf()
81
82 t = model.get('Solver.Time') * 1e3
83
84 m=3
85 n=3
86
87 ax = plt.subplot(m,n,1)
88 ax.plot(t, model.get('Model.C.p'), label='Chamber', color='k')
89 ax.set_title('Pressure')
90 plt.legend()
91
92 ax = plt.subplot(m,n,2)
93 ax.plot(t, model.get('Model.C.V'), label='Chamber', color='k')
94 plt.ylim([0, 1e-3])
95 ax.set_title('Volume')
96 plt.legend()
97
98 ax = plt.subplot(m,n,3)
99 ax.plot(t, model.get('Model.CiSy.q'))
100 ax.plot(t, model.get('Model.ChaSyArt.q'))
101 ax.plot(t, model.get('Model.SyVenCha.q'))
102 plt.ylim([0, 1e-3])
103
104 ax.axhline(0, color='k', linestyle='--')
105 ax.set_title('Flow')
106 plt.legend()
107
108
109 ax = plt.subplot(m,n,4)
110 ax.axhline(0, color='k', linestyle='--', lw=1)
111 ax.set_ylabel('C')
112 plt.legend()